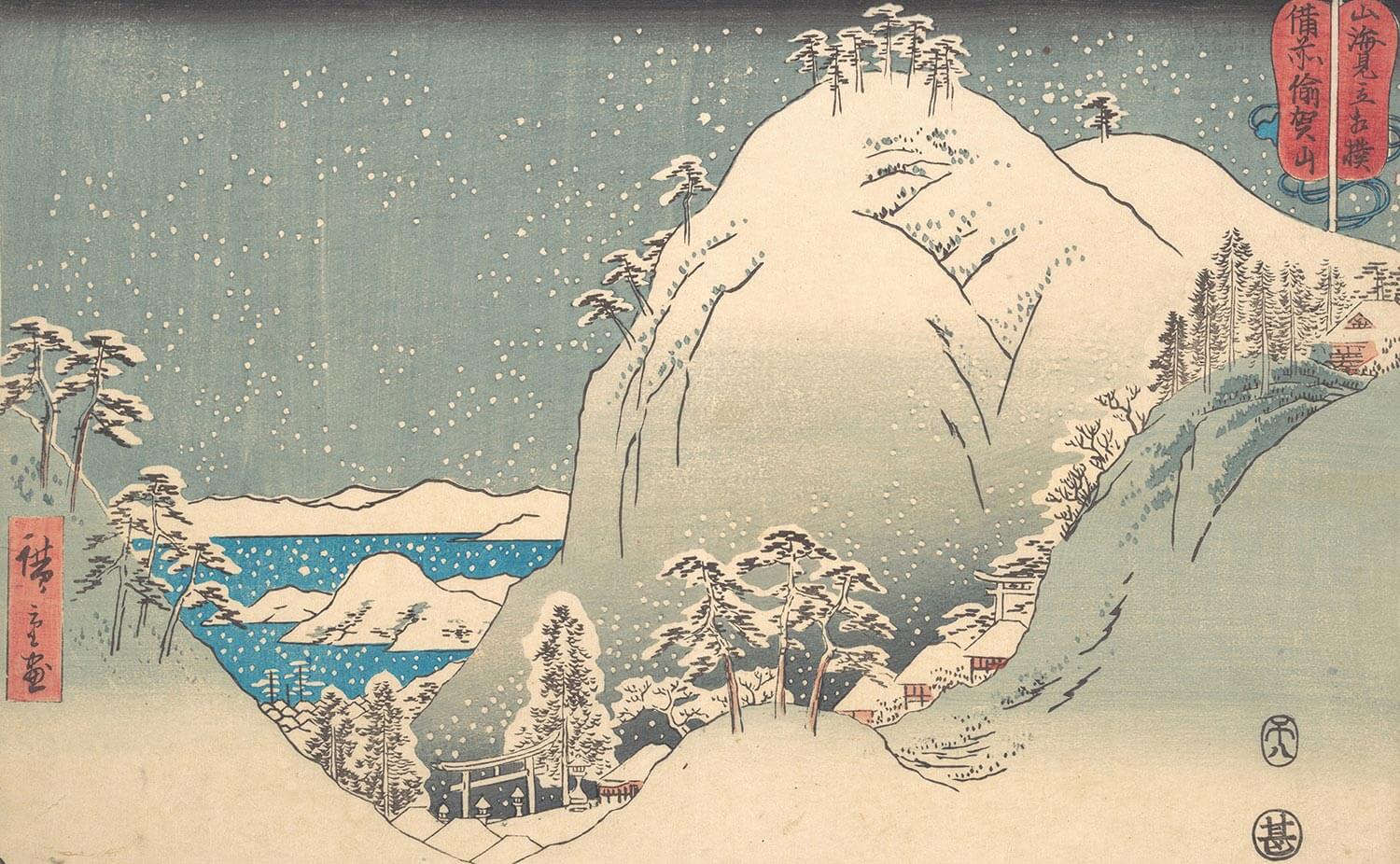
Add Swagger to .NET Core Project
Technology | C# |
---|---|
Editor of the Page | Latif Bahadır ALTUN |
Add package to project.
👨🏻💻Add these packages:Swashbuckle.AspNetCore
Swashbuckle.AspNetCore.Swagger
Swashbuckle.AspNetCore.SwaggerUI
Add code to Startup.cs
Add below code in Startup.cs ConfigureServices method:
var contact = new OpenApiContact() { Name = "ARD HEALTH", Email = "info@ardhealth.com", Url = new Uri("http://ardhealth.ardsistem.com.tr/") }; var info = new OpenApiInfo() { Version = "v1", Title = "PHE API", Description = "Rising costs of healthcare due to the ageing population and related increase of non-communicative diseases urges for finding ways to save expenses by diminishing the need for care, and making the current care more efficient. Current care provision is reactive and process driven, treating patients according to predefined pathways with limited possibilities to take into account the individual needs or abilities. Health authorities and care providers are finally noticing the one resource that had remained unused – the person or patient him/herself! By starting with the primary need of the person – to be healthy – and including him/her into the process in an active role, new paradigms for care become possible. Significant cost reductions can be achieved by preventive solutions to help the person adopt a healthy lifestyle – thus reducing the number of patients – and by providing the person with tools to actively participate in the treatment when diseases do arise – thus decreasing the burden on care personnel.", TermsOfService = new Uri("http://ardhealth.ardsistem.com.tr/"), Contact = contact }; services.AddSwaggerGen(c => { c.SwaggerDoc("v1", info); });
Add below code in Startup.cs Configure method:
app.UseSwagger(); app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "PHE API v1"); });
Hide Endpoints in Controllers
If you want hide all controller method and its controller:
[ApiExplorerSettings(IgnoreApi = true)] public class ActivityController : ControllerBase
If you want hide spesific method in controller:
[ApiExplorerSettings(IgnoreApi = true)] public ActionResult GetList()
Change launchSettings.json
"launchUrl": "swagger",
Add comment in SwaggerUI
Firstly, add below code in Startup.cs ConfigureServices method:
services.AddSwaggerGen(c => { c.SwaggerDoc("v1", info); // Set the comments path for the Swagger JSON and UI. var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml"; var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile); c.IncludeXmlComments(xmlPath); });
Then, add below code in<app.csproj>:
<PropertyGroup> <GenerateDocumentationFile>true</GenerateDocumentationFile> <NoWarn>$(NoWarn);1591</NoWarn> </PropertyGroup>
Then, go to Program.cs file and change it like below:
#pragma warning disable CS1591 public class Program { public static void Main(string[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); }); } #pragma warning disable CS1591
Finally, go to the controllers and its methods. Write like below:
/// <summary> /// Random Forest Learning endpoint. /// </summary> /// <remarks> /// The system receives a file with this endpoint. It provides learning with the ".csv" extension file. /// </remarks> /// <param> There is no parameter to enter this field. You need to post the file with csv extension in the request body.</param> /// <returns>Returns successful or incorrect message.</returns> /// <response code="200">Returns success.</response> /// <response code="400">Indicates an error in the extension or internal values of the uploaded file.</response> [HttpPost] public ActionResult PostFile()
Matters Needing Attention
❗If "Failed to fetch. Internal Server Error 'swagger / v1 / swagger.json' file not found." If the error is received, you can find a clear explanation of the error by going to the url "localhost: port / swagger / v1 / swagger.json".‼️Also, there should not be any functions that do not contain attributes such as [HttpGet], [HttpPost], [HttpPut], [HttpDelete] in the methods in your controller files. If it happens, the swagger returns an error. If there are functions that you do not use as endpoints in your controller functions and do not need the above attributes, please add the [NonAction] attribute above them.⁉️A Base class swagger used in Controller classes other than ControllerBase returns an error. No solution found.